So how was the program made?
This program doesn't detect age exactly but can provide approximate age. I am 21 years old, and the program shows I am 22, so there is only small difference. This program begins by loading the pre-trained model, which is stored in a file named age-model.h5. The predict_age function is responsible for preprocessing the input image by resizing it to the expected dimensions (224x224 pixels), normalizing the pixel values, and passing it through the model to obtain an age prediction.
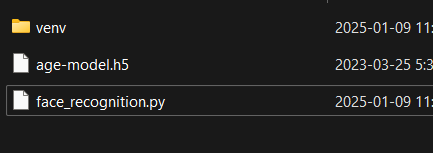
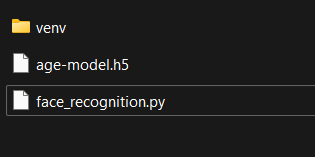
age-model.h5 is loaded through the line of code
model = load_model('age-model.h5', custom_objects={'mse': 'mean_squared_error'})

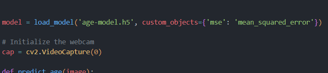
It is essential that TensorFlow, OpenCV, and numpy are installed before running script. To install them we can use following code in terminal. (I have used Vs Code for all of my programs)
pip install opencv-python
pip install numpy
pip install tensorflow
pip install h5py (if encountered loading .h5 file)
Now if everything is ready let me tell in short how the code in program is running
Load the Pre-trained Model:
Load the age-model.h5 file to predict age using load\_model.
2. Initialize Webcam:
Open the webcam feed using cv2.VideoCapture(0).
3. Define Age Prediction Function:
Resize the frame to 224x224 (model input size).
Normalize pixel values to [0, 1].
Predict the age using the model.
4. Capture Frames in a Loop:
Read each webcam frame using cap.read().
Pass the frame to the predict\_age() function.
5. Display Results:
Overlay the predicted age on the frame using cv2.putText.
Show the updated frame with cv2.imshow.
6. Exit on 'q' Key:
Exit the loop and close the webcam when 'q' is pressed.
Release resources and close all windows.
Below is the full code snippet
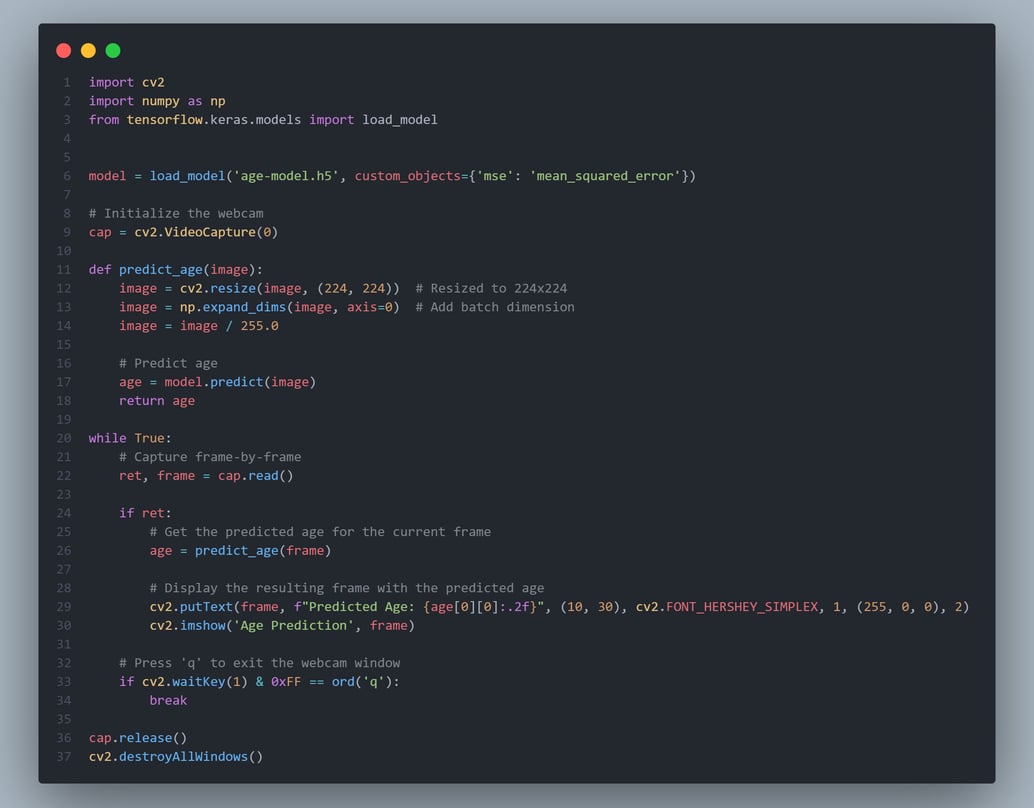
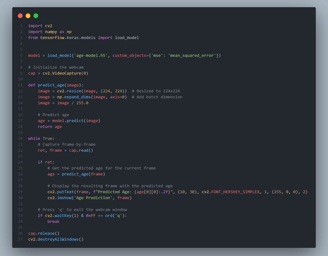