Steps for making python motion detector
Detailed breakdown
Computer vision
Computer vision involves enabling machines to interpret and analyze images or videos. In this code, the OpenCV library is used for face detection and motion detection.
Face detection
The code uses a pre-trained Haar Cascade classifier, a feature-based approach for detecting objects (in this case, faces) in images.
Motion detection
Motion detection is implemented here by analyzing the difference in the face's center position between consecutive frames.
Basics concepts
OpenCV2 is imported provides tools for image and video processing.
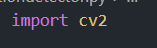
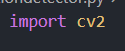
Loading the Pre-trained Haar Cascade Classifier


Initializing Webcam
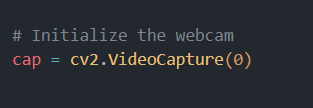
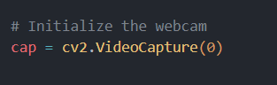
Storing Previous Face Position
These variables store the position of the center of the face detected in the previous frame for motion detection.
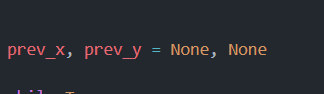
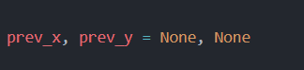
Reading Frames
cap.read(): Captures a frame from the webcam.
ret: Boolean indicating if the frame was successfully read.
frame: The captured image.
If ret is False, the loop exits, indicating no frame was captured.
Face detection


faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
detectMultiScale:
gray: The grayscale frame.
scaleFactor=1.1: Compensates for faces of different sizes (due to distance from the camera).
minNeighbors=5: Minimum neighbors required for a detection to be considered valid.
minSize=(30, 30): Minimum size of the detected face.
The output, faces, is a list of rectangles, each represented as (x, y, w, h):
(x, y): Top-left corner of the rectangle.
(w, h): Width and height of the rectangle.
Processing Detected Faces

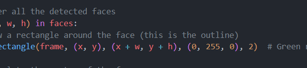
cv2.rectangle: Draws a rectangle around the face on the original frame.
(x, y): Top-left corner of the rectangle.
(x + w, y + h): Bottom-right corner of the rectangle.
(0, 255, 0): Green color in BGR format.
2: Thickness of the rectangle.
Detecting movements
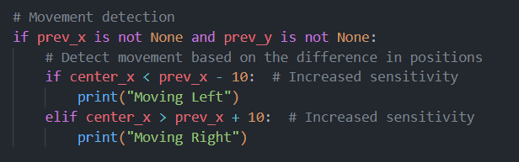
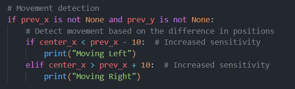
Compares the current face's center with the previous center (prev_x, prev_y).
If the difference exceeds a threshold (10 pixels):
Prints "Moving Left" or "Moving Right".
This creates a basic movement detection system.
Complete code snippet
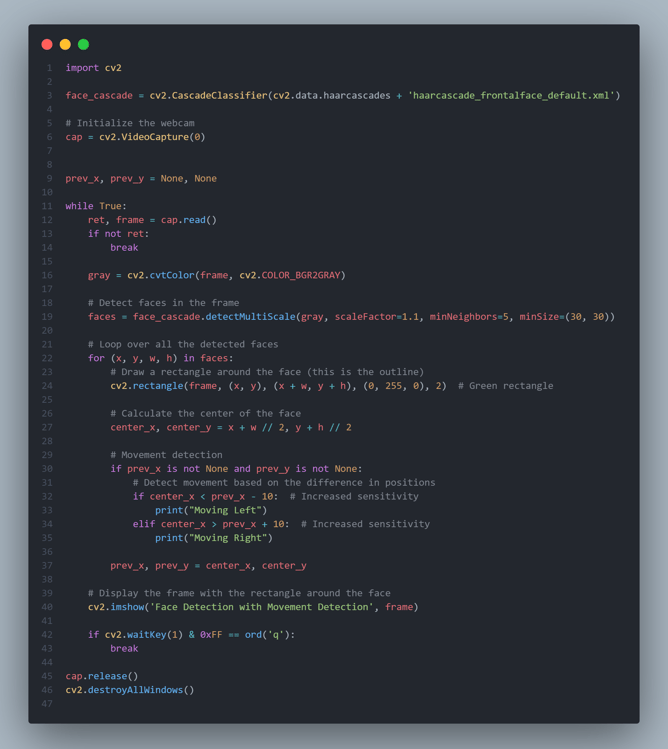
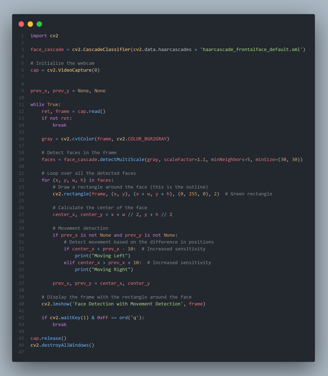